Real-time Web Product Scanning
One of the most common ways to integrate the EVRYTHNG Platform into a consumer product’s life cycle is to enable consumers to engage with the product (and its brand) through an on-pack trigger. Common examples include QR codes, DataMatrix codes, or the common GS1 UPC/EAN barcodes, which can be found on most products available in shops and supermarkets.
This kind of integration offers enormous benefits to both the brand and the consumer. Examples include insight into product origin, rewards, special offers, engagement metrics, and more. These benefits are made possible through the trigger’s ability to connect to the product’s online digital identity. A common approach to building this integration involves a brand-centric mobile experience delivered through a web-based application, such as a campaign page or the brand website. Examples of these engagement mechanisms include a "view provenance information" or "register to earn rewards" scenario.
An example application is shown below. It involves branding, a call to action, and a view through the camera viewfinder so that the user can aim the camera effectively:
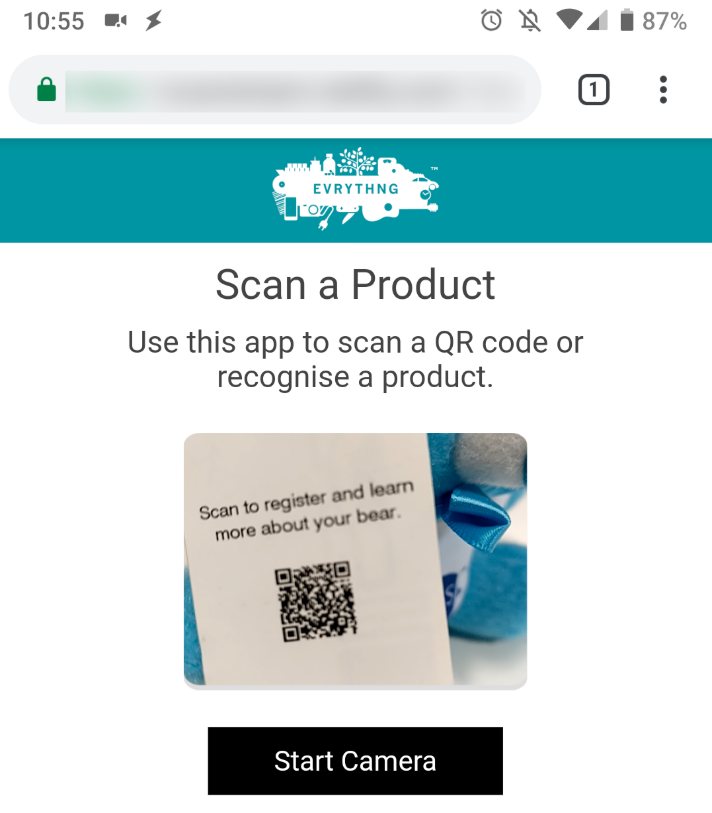
This sort of application is built with one of our web SDKs, scanthng.js, which lets developers to enhance their web apps with the ability to scan one of about 20 kinds of barcodes directly from a mobile web browser. With the scanthng.js, you can find out which EVRYTHNG product or Thng the barcode is associated with. This information can then power an experience with details about that product (for provenance, authenticity, or informative purposes) or perform a static or dynamic redirection to another brand page or resource. The benefits of using our SDK for this purpose are twofold:
-
No need to write code for scanning, uploading, matching products from scratch.
-
Automatic query and discovery of the full product data the barcode refers to.
This walkthrough shows you how easy it is to create a scanning experience that drives a deep level of engagement between a consumer and the brand.
Set up the account
Log into your EVRYTHNG account using the Dashboard. Create an account if you don't already have one. This is where all the data items are set up that power the redirection experience.
Choose a project from the Current project drop-down in the navigation pane, or create one. Projects are used to group resources according to their purpose. An example project is shown below:
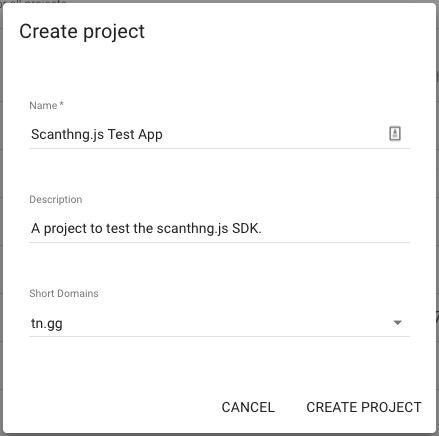
Create an application within this project using the Applications link in the navigation pane. Application resources represent external apps, including the web app that this walkthrough helps you create, by providing context and credentials (specifically API keys). An example is shown below:
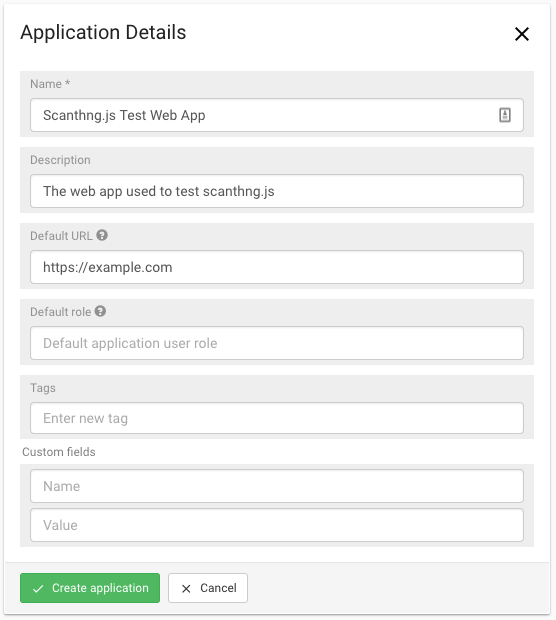
After the application has been created, you can copy the Application API Key that's used to authenticate the web scanning app.
Click Show key in the API Key section and click the copy button to securely copy the entire key. Store the key locally so it's available when you're ready to use it.
Set up the product
The web app you create in this walkthrough is used to scan a QR code located on a physical product. For testing purposes, you create a product resource in the EVRYTHNG platform to represent the physical item and to collect action data on it. In the case of scanning the QR code, actions are created each time it is scanned by a consumer. Actions also provide an opportunity to add a dynamic redirection depending on context items such as location, demographics, time, and so on, using the Redirector.
Scanthng.js generates an action of type initial scan
, which isn't directed to any project. To have the action recorded on your application, create a global redirector by clicking Redirector in the navigation pane and then clicking Create rule.
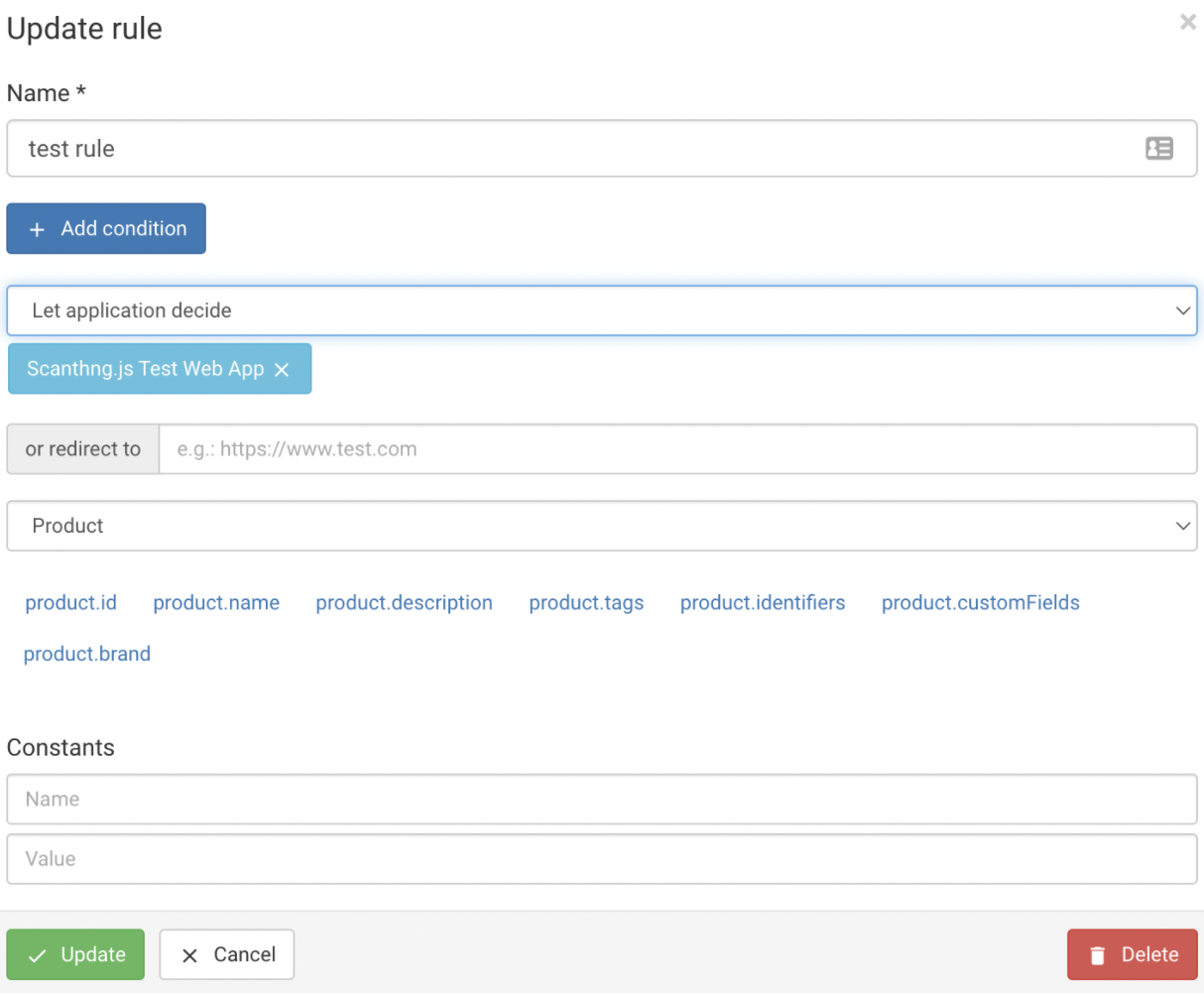
Leave the or redirect to text box blank. The global redirector now redirects the action to the Scanthng.js Test Web App
application.
Ensure the correct project is selected at the top. Create a product resource in the Products section of the navigation pane. The Application API Key can see the product by sharing the same project scope. An example product is shown below:
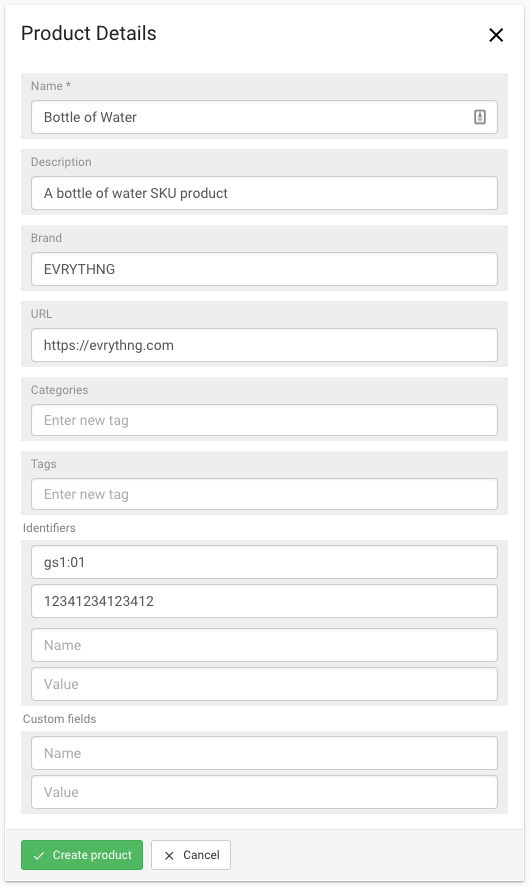
This example includes the gs1:01
(GS1 GTIN) value in its identifiers
. This lets you use the GS1 Digital Link standard and refer to the product using a URL that's readable by phones and point-of-sale systems.
The final step in the product setup is redirection. In the EVRYTHNG Platform, products and Thngs can be associated with a redirection to a static URL such as a product information or campaign page. The redirection can also be dynamic, as previously mentioned, using Redirector.
To set up the redirection for this product, click the product in the Products list. On the Product Details view, click Setup redirection. An example redirection is shown below, which describes the Short URL shown to consumers (as a link or a QR code) and the Redirect URL, where the user is eventually redirected.
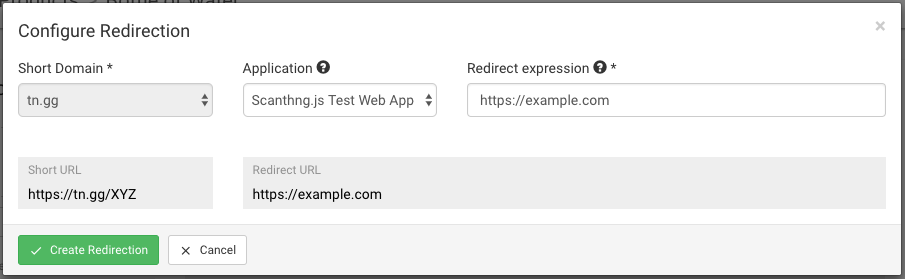
After the redirection is set up, test it by scanning the QR code shown on the Product Details page. You can use any available mobile barcode scanner app, a built-in feature such as Google Lens, or the iOS Camera application. If you include any of the available GS1 Digital Link identifiers, both the short URL QR code and a GS1 Digital Link QR code are shown for you to scan.
For added realism, add the product’s QR code to the example template bottle label below and wrap it around a bottle you have available. Doing so better emulates scanning the physical product for a consumer experience.
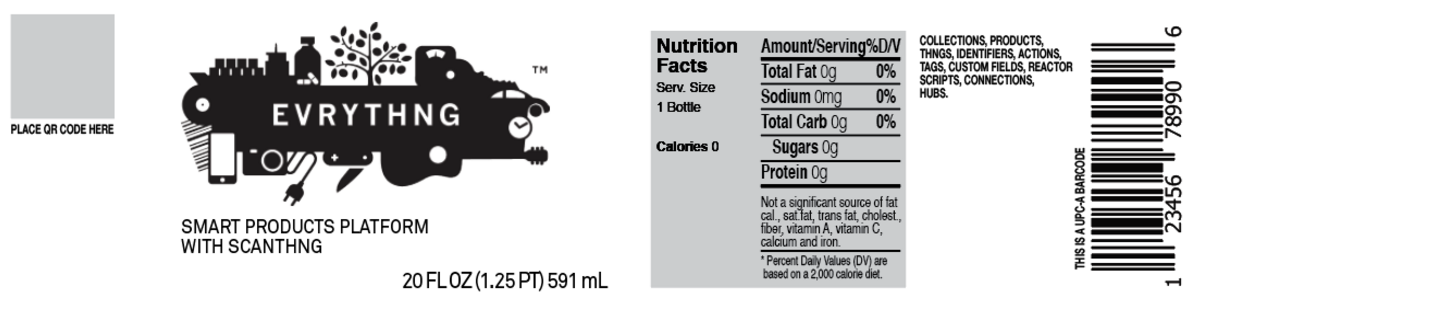
Set up a scanning web app
With the account setup complete, it is time to create a simple scanning web application that can be customized by the brand as required. It uses the scanthng.js
SDK to easily scan the barcode attached to the product and identify the full product in the EVRYTHNG Platform. In this walkthrough, we explain the general steps and present a finished implementation to use as an example.
The web app being developed must include the following dependencies as <script>
tags or local files:
-
evrythng.js - the EVRYTHNG JavaScript SDK that allows easy access to the resources in the account.
-
scanthng.js - a plugin for
evrythng.js
that adds the ability to scan barcodes and identify the products and Thngs that they are associated with. -
jsQR - dependency for
scanthng.js
that allows decoding of QR codes within the browser, removing the need for an external service.
This can be done by installing the three packages in the initialized npm directory: npm install --save evrythng jsqr scanthng
.
In the main project directory, create a folder named lib
and copy the file node_modules/jsqr/dist/jsQR.js
into the newly created folder.
The packages can now be imported into an HTML file:
<script src="./node_modules/evrythng/dist/evrythng.browser.js"></script>
<script src="./node_modules/scanthng/dist/scanthng.js"></script>
<script src="./lib/jsqr.js"></script>
When building a web app with evrythng.js
and its plugins, the first steps are to initialize the SDK and create a scope object that acts on behalf of that type of actor with the actor's API key. In this case, you initialize the SDK by including the scanthng.js
plugin and creating an Application
scope from which you can make API requests:
evrythng.setup({
apiVersion: 1 // legacy version
});
// Apply the plugin to the main SDK
evrythng.use(ScanThng);
const APPLICATION_API_KEY = 'YOUR_TRUSTED_API_KEY';
// Create scope for working with the application resource
const app = new evrythng.Application(APPLICATION_API_KEY);
This app
scope contains the methods used to perform scanning within the web app, and to retrieve the corresponding resources from the associated EVRYTHNG account.
The recommended way to scan barcodes and identify products within a web app is to use the scanStream()
function of scanthng.js
. This method uses a containing element in your web app to show a styled video stream and then attempts to decode QR codes at regular intervals using jsQR
. If one is found the Promise is resolved, and the results can be used as required to redirect or show an experience based on the data contained in the identified product or Thng. An example usage of this method is shown below for a web app that has a <div id="stream_container"/>
included:
// Scan for 2D QR codes with a video stream within the specified container
app.scanStream({
filter: { method: '2d', type: 'qr_code' },
containerId: 'stream_container',
})
.then(handleResults)
.catch(alert);
The handleResults
function receives an object that has a standard structure once a barcode is detected (the promise will be resolved). If the decoded QR code contained in any given stream frame is a short URL associated with a product or Thng, the results will contain the full object of that resource and a list of redirections available. If not, the meta
property will contain the decoded value of the QR code. This could still be a URL but not associated with an EVRYTHNG resource redirection, as is the case with most common QR codes already on packaged, consumer goods.
The code example shows one way these results could be used to populate an <a>
element that the app user could click on. This is shown in full in the complete example at the end:
const handleResults = (res) => {
const anchor = document.getElementById('result');
if (!res.length) {
// No results
anchor.innerHTML = 'No results';
return;
}
if (res.length && !res[0].results.length) {
// Raw URL
anchor.innerHTML = res[0].meta.value;
anchor.href = res[0].meta.value;
return;
}
// Full result with redirections
const url = res[0].results[0].redirections[0];
anchor.innerHTML = url;
anchor.href = url;
};
Besides 2D QR codes, this method can be used for other supported kinds of barcode, but the decoding happens using the EVRYTHNG API instead of within the browser. Depending on your use-case, this is likely still acceptable in order to scan DataMatrix codes from a video stream, for example.
To scan other barcode types with a video stream, change the method
and type
to those required as they appear in the documentation above for the required barcode type. For example, DataMatrix codes:
app.scanStream({
filter: { method: '2d', type: 'dm' },
containerId: 'stream_container',
})
.then(handleResults)
.catch(alert);
This then identifies all Thngs and products in scope of the authenticated application that have a corresponding value set for the dm
identifier.
Full code example
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Test Web Scanner</title>
</head>
<body>
<div id="stream_container">
<!-- video will be inserted here -->
</div>
<a id="result">
<!-- result will be inserted here -->
</a>
<script src="./node_modules/evrythng/dist/evrythng.browser.js"></script>
<script src="./node_modules/scanthng/dist/scanthng.js"></script>
<script src="./lib/jsqr.js"></script>
<script>
const APP_API_KEY = "YOUR_TRUSTED_API_KEY"; // trusted API key
evrythng.setup({
apiVersion: 1 // legacy version
});
// Apply the plugin to the main SDK
evrythng.use(ScanThng);
// Create scope for working with the application resource
const app = new evrythng.Application(APP_API_KEY);
const handleResults = (res) => {
// console.log(res)
const anchor = document.getElementById('result');
if (!res.length) {
// No results
anchor.innerHTML = 'No results';
return;
}
if (res.length && !res[0].results.length) {
// Raw URL
anchor.innerHTML = res[0].meta.value;
anchor.href = res[0].meta.value;
return;
}
// Full result with redirections
const url = res[0].results[0].redirections[0];
anchor.innerHTML = url;
anchor.href = url;
};
// Scan for 2D QR codes with a video stream within the specified container
app.scanStream({
filter: { method: '2d', type: 'qr_code' },
containerId: 'stream_container',
})
.then(handleResults)
.catch(console.log);
</script>
</body>
</html>
Conclusion
This walkthrough presented the potential benefits of enabling redirections for product digital identities and insight into how easy it can be to set up a simple consumer scanning experience. The example app provides a starting point for developing more complex applications for real-world brands and experiences.
Besides the redirection capabilities demonstrated here, the Redirector enhances a static redirection into one dynamically based on time, location, demographics, and so on.
You can see the complete source code for the example app that was explored in the scanthng-example
repository on GitHub. You can also see a more complex example that's useful for testing scanning applications in multiple projects here.
Updated over 1 year ago