Instrument with Actions
The actions API allows you to create discrete notifications or events attributed to action types that impart meaning to them. By creating actions of different action types, you can draw on some insights from the analytics provided in the EVRYTHNG Dashboard. In addition, actions have customFields
, allowing you to add any additional data about the user's behavior, page location, or errors encountered that might also be required.
This walkthrough shows a simple use case of actions and action types - instrumenting a web page or web application to record the frequency of different user interactions:
- When a page is loaded.
- When a button is clicked (that doesn't cause the browser to navigate away)
- When an error occurs.
Another page interaction not concerned with navigation.
To help implement this kind of application of actions as quickly as possible, we provide a special feature of the evrythng.js SDK for you to add to the web page or application to be instrumented.
Getting Started
The instrumentation conmprises the following process:
- Create an anonymous Application User to represent the visitor.
- Record an action of type
_PageVisited
, including the domain URL visited. - Make available a function
createAction()
that can create an action at any other point.
To create an Application User, you must first create a project and application using the Dashboard or API. After this is done, make a note of the Application API Key (not the Trusted Application API Key) for use later on.
Finally, create the _PageVisited
action type if it doesn't already exist (using the Dashboard or API), as well as any other types you'll use in instrumenting your web page. For example: _VideoWatched
, _UserLogin
, or _BasketCleared
. Be sure these action types are scoped to the same project, or they won't be available for the anonymous Application User to use.
Code Example
Add the following snippet to the <body>
of the web page(s) to be instrumented, with the following change:
- Set a value for
APPLICATION_API_KEY
with the Application API Key you noted earlier.
<!-- evrythng.js SDK -->
<script src="https://d10ka0m22z5ju5.cloudfront.net/js/evrythng/5.5.0/evrythng-5.5.0.js"></script>
<!-- Actions snippet -->
<script type="text/javascript">
// Create ActionApp scope using Application API Key
const APPLICATION_API_KEY = '';
var actionApp = new evrythng.ActionApp(APPLICATION_API_KEY);
// Record that a page has been visited
actionApp.pageVisited();
</script>
After the browser loads this snippet, three things occur:
- An anonymous Application User is created, and their Application User API Key is remembered for follow-up visits.
- An action of type
_PageVisited
is created, with the currentwindow.location.href
included incustomFields
. - A function
createAction()
is available from theactionApp
object for use anywhere it's required.
To create the additional actions to _PageVisited
, use the createAction()
function at the appropriate time.
Important
For each action type used, the corresponding action type resource must be created in the same project scope first, including the automatic
_PageVisited
action type.
const infoTab = document.getElementById('tab_info');
infoTab.addEventListener('click', (e) => {
const userId = localStorage.getItem('input_userId');
// Create an action including the user ID who logged in
actionApp.createAction('_InfoTabPressed', { userId });
});
Results
In this way, many user interactions or events can be tracked anonymously and the data shown on Dashboard widgets. For example:
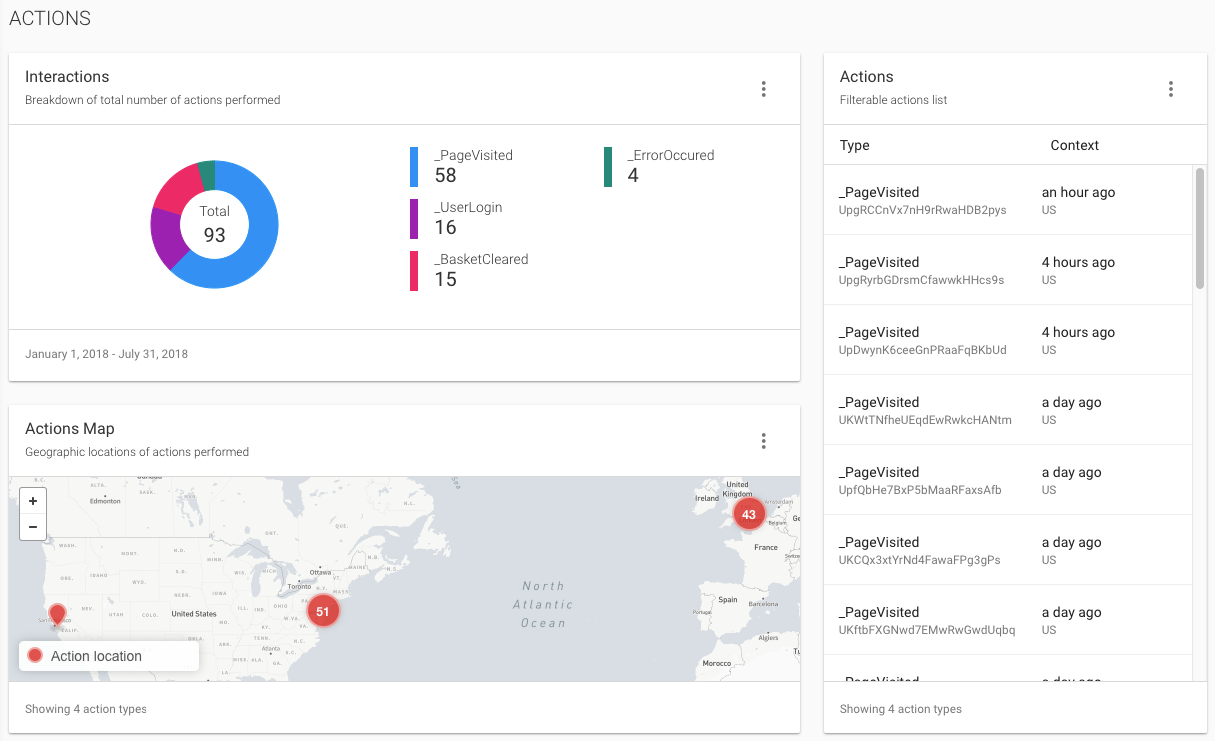
Updated over 1 year ago